Verify beneficiary details - name match
Confirming that beneficiary details match your customer
According to FATF, you should verify the accuracy of the beneficiary information you receive in the travel rule message:
This means that in addition to verifying that the address belongs to you, you should also compare the beneficiary's name (and additional information, if applicable) in the travel rule message against the KYC details of your customer that you collected and confirmed during the onboarding stage.
When to verify the beneficiary name
Usually, the beneficiary name matching would happen when you confirm that you control the wallet address. However, it can be done afterward as well (with some caveats):
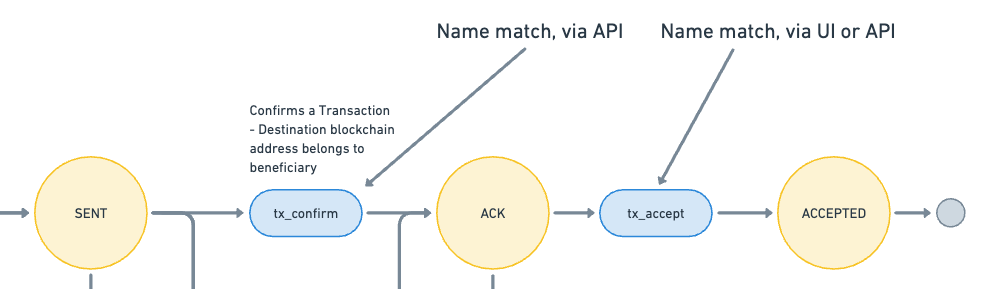
Name match via API
To perform Name match via API you have to:
- deactivate the auto-confirmation ;
- setup the notification webhook;
- when receiving an incoming transfer with SENT status and
beneficiaryDid
is your VASP did, retrieve PII data calling txInfo; - perform the beneficiary name match;
- call txConfirm or txReject depending on the outcome of the name match:
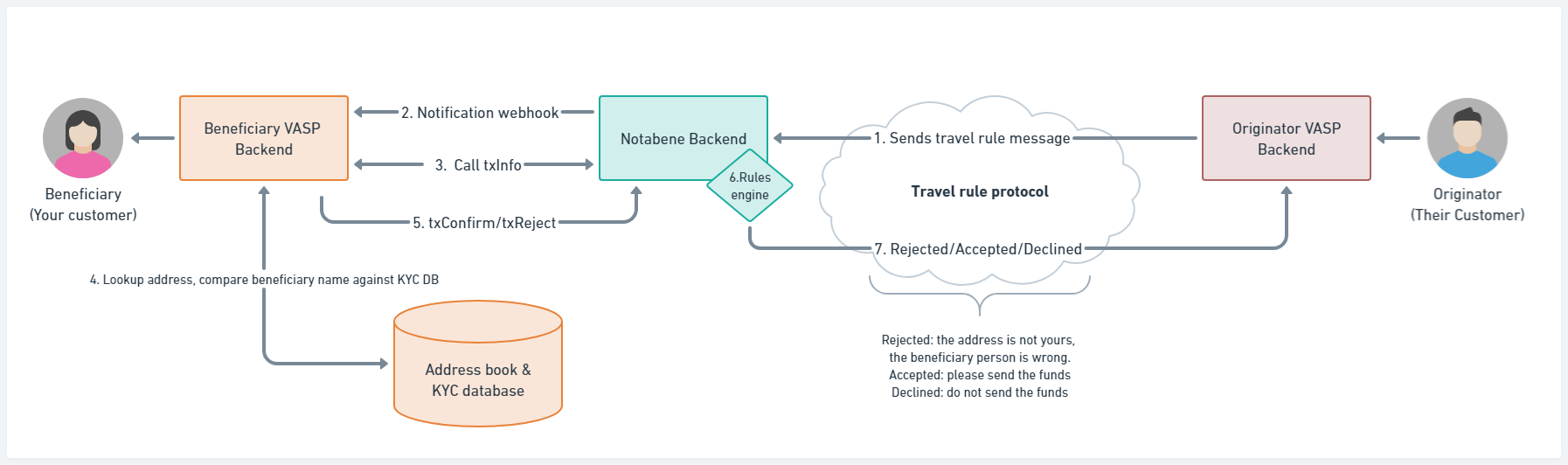
Name match via UI
Note that if you want to perform name matching using the Notabene dashboard, you cannot have Accept rules active as they would run immediately once the status hits ACK.
Name match example
Using fuzzy logic to compare:
"beneficiaryPersons": [
{
"naturalPerson": {
"name": [
{
"nameIdentifier": [
{
"primaryIdentifier": "Heller",
"secondaryIdentifier": "Emory"
}
Notabene VASP SG Customer Database v1.234
Customer ID: 123456789
Wallet addr: 3uyZiAC2khxuxC26weZcrcEWubE8vEjY3
Name: Emory Heller
DoB: 01/01/1999
...
Because the name you receive can be written in different ways, using fuzzy matching might be the best way to compare the information:
from fuzzywuzzy import fuzz
customerName = "Emory Way Heller"
primaryIdentifier = "Heller"
secondaryIdentifier = "Emory"
mergedIdentifier = primaryIdentifier+secondaryIdentifier
Ratio = fuzz.ratio(customerName.lower().replace(" ", ""),mergedIdentifier.lower().replace(" ", ""))
print(Ratio)
Ratio:88
#You can also use fuzz.token_sort_ratio to cater for mixed-up first and last name:
#In token sort ratio, the strings are tokenized and pre-processed by converting to lower case and getting rid of punctuation. The strings are then sorted alphabetically and joined together. Post this, the Levenshtein distance similarity ratio is calculated between the strings.
Suppose the address is yours and the beneficiary matches your customer. In that case, you should call txConfirm to change the status from SENT
to ACK
and receive the originator information in the travel rule message for sanctions screening.
Ratio = 88
fuzzyMinimum = 87
my_wallets = ["39oSD4x8a9EBb1Ft7weNFbqhZZuz", "3uyZiAC2khxuxC26weZcrcWubE8vEjY3"]
destination = "3uyZiAC2khxuxC26weZcrcWubE8vEjY3"
if (destination in my_wallets):
if Ratio > fuzzyMinimum:
txConfirm = requests.request("POST", url1, headers=headers1, data=payload1)
print("txConfirm")
else:
txDecline = requests.request("POST", url2, headers=headers2, data=payload2)
print("txDecline")
else:
txReject = requests.request("POST", url3, headers=headers3, data=payload3)
print("txReject")
Updated 5 days ago